Last Updated on March 19, 2021
If you want to programmatically retrieve the AWS regions one of the best ways to do this is via Python boto3 package.
In boto3, specifically the EC2 client, there is a function named describe_regions
. This will retrieve the regions that are in AWS.
See different uses of the describe_regions
function below.
- Basic code for retrieving the AWS Regions using boto3
- Listing All AWS Regions regardless if enabled or not
- Region OptInStatus Explained
- Getting the Region Code only (us-east-1, eu-central-1)
- Returning only specific Regions
Basic code for retrieving the AWS Regions using boto3
Here is a basic code for getting the AWS Regions in boto3.
import boto3
ec2_client = boto3.client('ec2')
response = ec2_client.describe_regions()
print(response)
response
is a dictionary that contains all active regions in the AWS Account.
The output of the Python script above can be seen below. I decided to format the output for easier reading, and show the whole response
to easily understand how to manipulate the output.
{
'Regions': [
{
'Endpoint': 'ec2.eu-north-1.amazonaws.com',
'RegionName': 'eu-north-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-south-1.amazonaws.com',
'RegionName': 'ap-south-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-west-3.amazonaws.com',
'RegionName': 'eu-west-3',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-west-2.amazonaws.com',
'RegionName': 'eu-west-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-south-1.amazonaws.com',
'RegionName': 'eu-south-1',
'OptInStatus': 'opted-in'
},
{
'Endpoint': 'ec2.eu-west-1.amazonaws.com',
'RegionName': 'eu-west-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-northeast-3.amazonaws.com',
'RegionName': 'ap-northeast-3',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-northeast-2.amazonaws.com',
'RegionName': 'ap-northeast-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-northeast-1.amazonaws.com',
'RegionName': 'ap-northeast-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.sa-east-1.amazonaws.com',
'RegionName': 'sa-east-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ca-central-1.amazonaws.com',
'RegionName': 'ca-central-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-southeast-1.amazonaws.com',
'RegionName': 'ap-southeast-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-southeast-2.amazonaws.com',
'RegionName': 'ap-southeast-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-central-1.amazonaws.com',
'RegionName': 'eu-central-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-east-1.amazonaws.com',
'RegionName': 'us-east-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-east-2.amazonaws.com',
'RegionName': 'us-east-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-west-1.amazonaws.com',
'RegionName': 'us-west-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-west-2.amazonaws.com',
'RegionName': 'us-west-2',
'OptInStatus': 'opt-in-not-required'
}
],
'ResponseMetadata': {
'RequestId': 'adace754-e32e-9265-b0d2-5c56f4405711',
'HTTPStatusCode': 200,
'HTTPHeaders': {
'x-amzn-requestid': 'adace754-e32e-9265-b0d2-5c56f4405711',
'cache-control': 'no-cache, no-store',
'strict-transport-security': 'max-age=31536000; includeSubDomains',
'content-type': 'text/xml;charset=UTF-8',
'content-length': '3875',
'vary': 'accept-encoding',
'date': 'Fri, 19 Mar 2021 01:59:53 GMT',
'server': 'AmazonEC2'
},
'RetryAttempts': 0
}
}
If you noticed that not all regions are listed in the above response (18 out of 21). The reason for this is that my AWS Account has not enabled all regions.
You can see the list of enabled AWS Regions in your account in My Account of your AWS Console. You can check the Managing AWS Regions documentation for more information.
Listing All AWS Regions regardless if enabled or not
To list all of the AWS Regions regardless if you have enabled the region or not you can use the AllRegions=True
parameter.
import boto3
ec2_client = boto3.client('ec2')
response = ec2_client.describe_regions(AllRegions=True)
print(response)
Below is the full response that will be displayed.
{
'Regions': [
{
'Endpoint': 'ec2.af-south-1.amazonaws.com',
'RegionName': 'af-south-1',
'OptInStatus': 'not-opted-in'
},
{
'Endpoint': 'ec2.eu-north-1.amazonaws.com',
'RegionName': 'eu-north-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-south-1.amazonaws.com',
'RegionName': 'ap-south-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-west-3.amazonaws.com',
'RegionName': 'eu-west-3',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-west-2.amazonaws.com',
'RegionName': 'eu-west-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-south-1.amazonaws.com',
'RegionName': 'eu-south-1',
'OptInStatus': 'opted-in'
},
{
'Endpoint': 'ec2.eu-west-1.amazonaws.com',
'RegionName': 'eu-west-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-northeast-3.amazonaws.com',
'RegionName': 'ap-northeast-3',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-northeast-2.amazonaws.com',
'RegionName': 'ap-northeast-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.me-south-1.amazonaws.com',
'RegionName': 'me-south-1',
'OptInStatus': 'not-opted-in'
},
{
'Endpoint': 'ec2.ap-northeast-1.amazonaws.com',
'RegionName': 'ap-northeast-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.sa-east-1.amazonaws.com',
'RegionName': 'sa-east-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ca-central-1.amazonaws.com',
'RegionName': 'ca-central-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-east-1.amazonaws.com',
'RegionName': 'ap-east-1',
'OptInStatus': 'not-opted-in'
},
{
'Endpoint': 'ec2.ap-southeast-1.amazonaws.com',
'RegionName': 'ap-southeast-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.ap-southeast-2.amazonaws.com',
'RegionName': 'ap-southeast-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.eu-central-1.amazonaws.com',
'RegionName': 'eu-central-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-east-1.amazonaws.com',
'RegionName': 'us-east-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-east-2.amazonaws.com',
'RegionName': 'us-east-2',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-west-1.amazonaws.com',
'RegionName': 'us-west-1',
'OptInStatus': 'opt-in-not-required'
},
{
'Endpoint': 'ec2.us-west-2.amazonaws.com',
'RegionName': 'us-west-2',
'OptInStatus': 'opt-in-not-required'
}
],
'ResponseMetadata': {
'RequestId': '1aab6d6f-6d87-090d-9df8-3c72b956d1d',
'HTTPStatusCode': 200,
'HTTPHeaders': {
'x-amzn-requestid': '1aab6d6f-6d87-090d-9df8-3c72b956d1d',
'cache-control': 'no-cache, no-store',
'strict-transport-security': 'max-age=31536000; includeSubDomains',
'content-type': 'text/xml;charset=UTF-8',
'content-length': '4689',
'vary': 'accept-encoding',
'date': 'Fri, 19 Mar 2021 02:50:44 GMT',
'server': 'AmazonEC2'
},
'RetryAttempts': 0
}
}
Region OptInStatus Explained
If you noticed that there are three values for the OptInStatus – opt-in-not-required
, opted-in
, and not-opted-in
.
opt-in-not-required
is the Opt In Status for default regions. This regions are enabled by default and cannot be disabled.
Below is an example of a region with a opt-in-not-required
OptInStatus.
{
'Endpoint': 'ec2.eu-west-1.amazonaws.com',
'RegionName': 'eu-west-1',
'OptInStatus': 'opt-in-not-required'
}
opted-in
is the status of a region that are not part of the default regions and was enabled in the regions section of AWS My Account. See example below.
{
'Endpoint': 'ec2.eu-south-1.amazonaws.com',
'RegionName': 'eu-south-1',
'OptInStatus': 'opted-in'
}
not-opted-in
is the status of the regions that are disabled in your AWS Account. See example below.
{
'Endpoint': 'ec2.me-south-1.amazonaws.com',
'RegionName': 'me-south-1',
'OptInStatus': 'not-opted-in'
}
In my AWS Account here are the list of enabled and disabled regions.
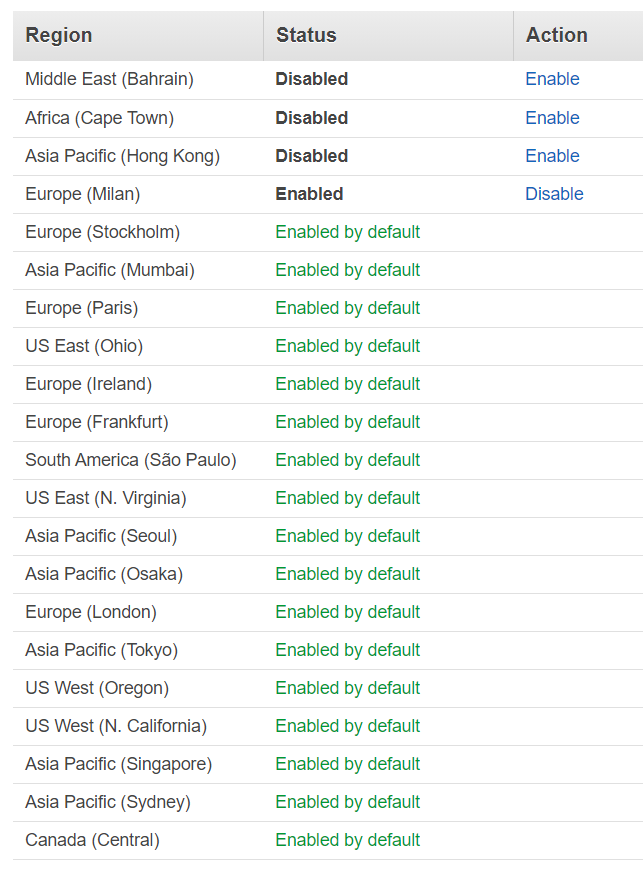
I enabled the Europe (Milan) – eu-south-1 region only for this blog post and I noticed that it will not immediately show when you call ec2_client.describe_regions()
. It took around 15 minutes before it showed with a status of opted-in
.
Getting the Region Code only (us-east-1
, eu-central-1
)
If the goal is to get only the Region Code such as ap-south-east-1
or us-west-2
, we will need to get the RegionName
of each region. To do this see the code below.
import boto3
ec2_client = boto3.client('ec2')
response = ec2_client.describe_regions()
regions = response['Regions']
for region in regions:
print(region['RegionName'])
The output will be below.
eu-north-1
ap-south-1
eu-west-3
eu-west-2
eu-south-1
eu-west-1
ap-northeast-3
ap-northeast-2
ap-northeast-1
sa-east-1
ca-central-1
ap-southeast-1
ap-southeast-2
eu-central-1
us-east-1
us-east-2
us-west-1
us-west-2
Returning only specific Regions
We can also limit the regions that we want to get by using the RegionNames
parameter.
The RegionNames
parameter needs a list of strings with values of the region code that you only want to return.
Below is a Python script that will fetch only the regions Sydney (ap-southeast-2), Milan (eu-south-1) and Cape Town (af-south-1).
import boto3
ec2_client = boto3.client('ec2')
response = ec2_client.describe_regions(
RegionNames=[
'ap-southeast-2',
'eu-south-1',
'af-south-1'
]
)
regions = response['Regions']
for region in regions:
print(region)
The output will be below.
{'Endpoint': 'ec2.af-south-1.amazonaws.com', 'RegionName': 'af-south-1', 'OptInStatus': 'not-opted-in'}
{'Endpoint': 'ec2.ap-southeast-2.amazonaws.com', 'RegionName': 'ap-southeast-2', 'OptInStatus': 'opt-in-not-required'}
{'Endpoint': 'ec2.eu-south-1.amazonaws.com', 'RegionName': 'eu-south-1', 'OptInStatus': 'opted-in'}
If you want to learn more about listing the AWS regions using boto3 you can check the references below.
boto3 documentation – Describe Amazon EC2 Regions and Availability Zones
We hope this helps you retrieve the AWS Regions using Python boto3. If you have questions, comments or suggestions let me know on the comments below.